How to add custom placeholders to WooCommerce email subject and body
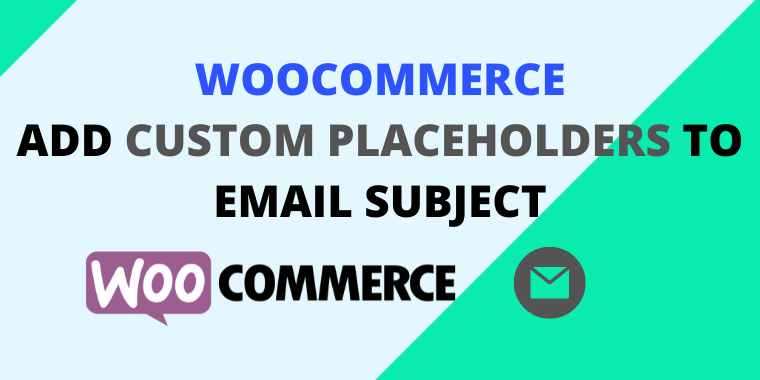
Table of Contents
- Default WooCommerce email placeholders
- How to create custom email placeholders
- How to use the custom WooCommerce email subject placeholders
It’s not uncommon for WooCommerce store owners to want to add their custom email placeholders in the subject and the body of the automated email that the WooCommerce store sends. By default, there are a limited amount of inbuilt email placeholders in WooCommerce.
Default WooCommerce email placeholders
Before you create your placeholders, make sure to check the existing WooCommerce email placeholders.
{site_title}
: The title of your WordPress site.{order_date}
: The date the order was made.{order_number}
: The unique order number.{customer_first_name}
: The first name of the customer.{customer_last_name}
: The last name of the customer.{customer_username}
: The username of the customer.{customer_email}
: The email address of the customer.{customer_note}
: The note added by the customer during checkout.{order}
: The entire order details.{billing_first_name}
: The first name of the person to whom the bill is addressed.{billing_last_name}
: The last name of the person to whom the bill is addressed.{billing_company}
: The company name to which the bill is addressed.{billing_address_1}
: The first line of the billing address.{billing_address_2}
: The second line of the billing address.{billing_city}
: The city of the billing address.{billing_state}
: The state of the billing address.{billing_postcode}
: The postal code of the billing address.{billing_country}
: The country of the billing address.{shipping_first_name}
: The first name of the person to whom the order is shipped.{shipping_last_name}
: The last name of the person to whom the order is shipped.{shipping_company}
: The company name to which the order is shipped.{shipping_address_1}
: The first line of the shipping address.{shipping_address_2}
: The second line of the shipping address.{shipping_city}
: The city of the shipping address.{shipping_state}
: The state of the shipping address.{shipping_postcode}
: The postal code of the shipping address.{shipping_country}
: The country of the shipping address.
Please note that the availability of these placeholders may depend on the specific email template you are using.
How to create custom email placeholders
To create custom placeholders to your WooCommerce email subject and body, we have to use one of the WooCommerce filter hooks woocommerce_email_format_string
. You will need some PHP knowledge to customize the code snippet to suit your needs. If you need support, feel free to contact our WordPress support.
/**
* @snippet Add custom placeholders to WooCommerce email subject and body
* @author WP Authors
* @compatible WooCommerce 3.2+
* @donate https://www.buymeacoffee.com/wpauthors
* @param [string] $string
* @param [object] $email
* @return string
*/
function wpa_filter_email_format_string( $string, $email ) {
// Get WC_Order object from email
$order = $email->object;
// Add new placeholders
$new_placeholders = array(
'{_first_name}' => $order->get_billing_first_name(),
'{_order_total}' => $order->get_total(),
'{_payment_method}' => $order->get_payment_method_title(),
);
// return the string with new placeholder replacements
return str_replace( array_keys( $new_placeholders ), array_values( $new_placeholders ), $string );
}
add_filter( 'woocommerce_email_format_string' , 'wpa_filter_email_format_string', 20, 2 );
In this example code, I have created three custom placeholders. _first_name
, _order_total
and _payment_method
. As per your requirement, you can add as many as placeholders you need. You can find the full list of available methods of the WooCommerce order object here. I used “_” underscore at the beginning of the placeholder to avoid potential conflicts.
How to use the custom WooCommerce email subject placeholders
There is no magic. You can follow the same steps just like a regular placeholder.
- Navigate to WooCommerce > Settings > Emails and select the email that you need to add the placeholder.
- Find the subject field and combine your subject with your new email subject placeholders.
Ex new order notification: New order from {_first_name} via {_payment_method}